Lint Filters And Testing
I don’t know about the lint filter on your dryer, but our current dryer, and every dryer I’ve used before this, had a message on or near the lint filter something like this.
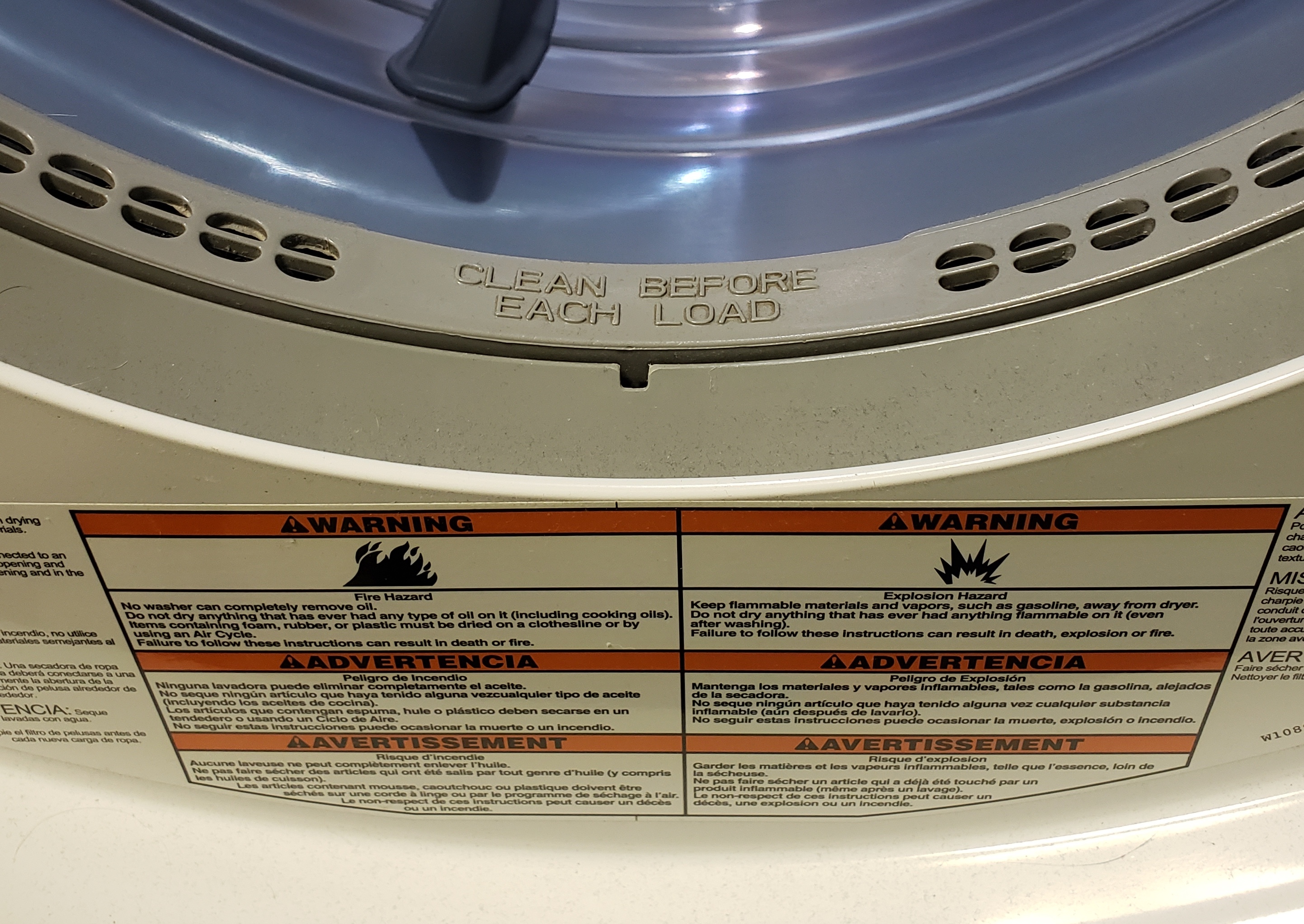
CLEAN BEFORE EACH LOAD
That’s pretty good advice. Sure, you should clean the filter after you use it. It’s polite and you want to be helpful to the next person who uses the dryer, but that doesn’t always happen. As a user of the washer and dryer, it’s your responsibility to make sure that they are ready for your use. That means enough soap, softener (if you use it), bleach (if you want), and cleaning the lint filter.
It’s the same way with software. You should always endeavor to leave things the way you found them. Return unused resources. Clean up any temporary files. Reset shared state. In short, you want to eliminate any unintended side effects and make your system as idempotent as possible. You don’t want the current run to be unduly influenced by the previous run. The thing is, no matter how hard you try, you can’t be sure the code you have at the end runs successfully to completion.
Which means, before you do something, you need to make sure things are set up the way you need them to be. Especially if it’s a repetitive task. Like a unit or TDD test. You run them all the time. Potentially multiple times an hour. And sometimes they fail in the middle. So they need to be idempotent and side-effect free.
There’s a name for this pattern. It’s the Arrange-Act-Assert (AAA) pattern. Do all your setup. Everything from ensuring the (virtual) file system is in the right state to setting up the environment to building and seeding a database. Then make the ONE call that does the thing you’re testing. Finally, do as many asserts as you need to ensure that things went the way you expected.
The AAA pattern is that simple. Arrange things how they should be. Act on the system. Assert everything came out as expected. Like everything else though, in practice there are nuances and complexities. In many cases there’s a part of Arrange that you want to run once before any test, but not before every test. You might need to assert that the Arrange step actually arranged things correctly. You’ll find that a lot of the Arrange code should be re-used. You probably want to clean up after the Assert. And there might be a cleanup that runs after all tests are done.
Then there are the efficiency temptations. If you’re doing a CRUD test you’ll probably be tempted to do it in one test. After all, the Arrange for Read, Update, and Delete is Create, so why do it separately? It’s faster and less code to do it in one test right? Probably not. It might be faster on initial write, but over time, as the code changes, that unnatural coupling is going to slow you down. So don’t do it. Remember, Act is doing the one call that you’re going to test. If you have multiple Acts in your test you should split it up into multiple tests.
And don’t forget to clean the dryer lint filter before each load.